cloudNet@ 팀의 가시다 님이 진행하는 테라폼 102 스터디 입니다. (테라폼으로 시작하는 IaC” (한빛미디어) 도서로 진행!)
- EC2 웹 서버 배포시 Apache 프로비저닝 하기
provider "aws" {
region = "ap-northeast-2"
}
resource "aws_instance" "example" {
ami = "ami-00d253f3826c44195"
instance_type = "t2.micro"
vpc_security_group_ids = [aws_security_group.instance.id]
user_data = <<-EOF
#!/bin/bash
yum install -y httpd
systemctl start httpd
echo "Hello, T101 Study" > /var/www/html/index.html
EOF
user_data_replace_on_change = true
tags = {
Name = "Single-WebSrv"
}
}
resource "aws_security_group" "instance" {
name = var.security_group_name
ingress {
cidr_blocks = ["0.0.0.0/0"]
from_port = 0
to_port = 0
protocol = "-1"
}
egress {
cidr_blocks = ["0.0.0.0/0"]
from_port = 0
to_port = 0
protocol = "-1"
}
}
variable "security_group_name" {
description = "The name of the security group"
type = string
default = "terraform-example-instance"
}
output "public_ip" {
value = aws_instance.example.public_ip
description = "The public IP of the Instance"
}
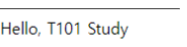
- AWS S3/DynamoDB 백엔드 구성
# AWS 프로바이더 설정
provider "aws" {
# 리전 'ap-northeast-2'(서울) 설정
region = "ap-northeast-2"
}
# S3 버킷 생성 리소스 정의
resource "aws_s3_bucket" "mys3bucket" {
# 버킷 이름 설정(minhotest-t101study-tfstate 예시)
bucket = "minhotest-t101study-tfstate"
}
# S3 버킷 버전 관리 리소스 정의
resource "aws_s3_bucket_versioning" "mys3bucket_versioning" {
# 생성된 버킷의 아이디 사용
bucket = aws_s3_bucket.mys3bucket.id
# 버전 관리 구성 블록
versioning_configuration {
# 버전 관리 활성화
status = "Enabled"
}
}
# 출력 값(S3 버킷 ARN) 정의
output "s3_bucket_arn" {
# 생성된 버킷의 ARN 사용
value = aws_s3_bucket.mys3bucket.arn
# 출력 값 설명 설정
description = "The ARN of the S3 bucket"
}
# DynamoDB 테이블 생성 리소스 정의
resource "aws_dynamodb_table" "mydynamodbtable" {
# 테이블 이름 설정
name = "terraform-locks"
# 결제 방식 설정: PAY_PER_REQUEST (요청 당 지불)
billing_mode = "PAY_PER_REQUEST"
# 해시 키 설정
hash_key = "LockID"
# 속성 설정
attribute {
# 속성 이름 설정
name = "LockID"
# 속성 유형 설정: 'S' (문자열 저장)
type = "S"
}
}
# 출력 값(DynamoDB 테이블 이름) 정의
output "dynamodb_table_name" {
# 생성된 테이블의 이름 사용
value = aws_dynamodb_table.mydynamodbtable.name
# 출력 값 설명 설정
description = "The name of the DynamoDB table"
}
----
terraform init && terraform apply
확인 후 yes 입력

위와 같이 S3 와 DynamoDB 확인을 하였습니다. 그다음 신규 디렉토리 만든 후 테라폼을 이용하여 test 하겠습니다.
cat <<EOT > backend.tf
# Terraform 설정
terraform {
# AWS S3를 백엔드로 사용하여 상태 파일을 저장하는 구성
backend "s3" {
# 상태 파일을 저장할 S3 버킷의 이름 (예: '$NICKNAME-t101study-tfstate')
bucket = "minhotest-t101study-tfstate"
# 상태 파일 저장될 디렉토리와 파일 이름 (예: '3.2/terraform.tfstate')
key = "3.2/terraform.tfstate"
# 상태 파일이 저장될 AWS 리전 (예: 'ap-northeast-2'(서울))
region = "ap-northeast-2"
# 상태 파일 잠금을 위해 사용할 DynamoDB 테이블 이름 (예: 'terraform-locks')
# 실행 중 동시 업데이트를 방지하기 위한 목적으로 활용
dynamodb_table = "terraform-locks"
# (주석 처리된 코드) 상태 파일의 암호화를 설정하는 부분
# encrypt 값을 'true'로 설정하면, 상태 파일을 서버 측 암호화로 암호화
# encrypt true
}
}
EOT
# S3 버킷에 파일 확인
# DynamoDB에 LockID 확인
terraform init && terraform apply
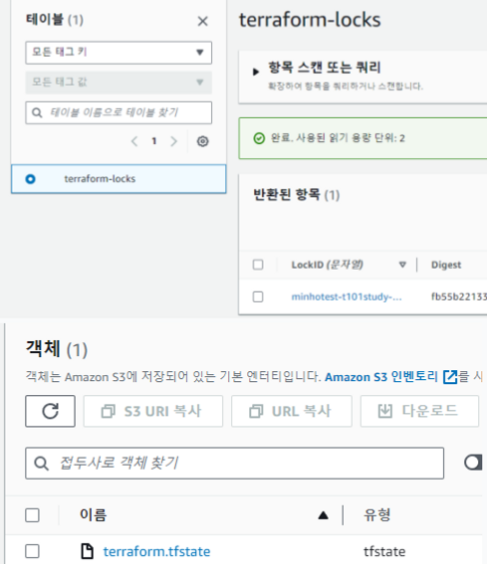
실습 완료되었다면 삭제 하자
#ec2 리소스 삭제
terraform destroy -auto-approve
# S3 버킷에 객체 삭제
# 해당 디렉토리 이동합니다.
aws s3 rm s3://$NICKNAME-t101study-tfstate --recursive
# S3 버킷에 버저닝 객체 삭제
aws s3api delete-objects \
--bucket $NICKNAME-t101study-tfstate \
--delete "$(aws s3api list-object-versions \
--bucket "${NICKNAME}-t101study-tfstate" \
--output=json \
--query='{Objects: Versions[].{Key:Key,VersionId:VersionId}}')"
# S3 버킷에 삭제마커 삭제
aws s3api delete-objects --bucket $NICKNAME-t101study-tfstate \
--delete "$(aws s3api list-object-versions --bucket "${NICKNAME}-t101study-tfstate" \
--query='{Objects: DeleteMarkers[].{Key:Key,VersionId:VersionId}}')"
# 백엔드 리소스 삭제
tfstate-backend$ terraform destroy -auto-approve
- lifecycle의 precondition 실습 내용에서 step0.txt ~ step6.txt 총 7개의 파일 이름 중 하나가 일치 시 검증 조건 만족으로 코드 작성
variable "file_name" {
default = "step0.txt"
}
resource "local_file" "abc" {
content = "lifecycle - step 0-6"
filename = "${path.module}/${var.file_name}"
lifecycle {
precondition {
#contains 함수는 특정 값이 목록 안에 있는지 확인하는 Terraform 함수입니다. 만약 값이 목록에 있다면 true를 반환하고, 없다면 false를 반환
condition = contains (["step0.txt", "step1.txt", "step2.txt", "step3.txt", "step4.txt", "step5.txt", "step6.txt"],var.file_name)
error_message = "file name should be in the range: step0.txt to step6.txt"
}
}
}